Chapter 7 Categorical Data Analysis
Everything analysis covered so far has used a continuous variable as a target variable of interest. What if our target variable was categorical instead of continuous? Our analysis must change to adjust.
This Chapter aims to answer the following questions:
How do you explore categorical variables?
- Nominal vs. Ordinal
- Tests of Association
- Measures of Association
How do you model a categorical target variable?
- Logistic Regression
- Interpreting Logistic Regression
- Assessing Logistic Regression
7.1 Describing Categorical Data
We need to first explore our data before building any models to try and explain/predict our categorical target variable. With categorical variables, we can look at the distribution of the categories as well as see if this distribution has any association with other variables. For this analysis we are going to still use our Ames housing data. Imagine you worked for a real estate agency and got a bonus check if you sold a house above $175,000 in value. Let’s create this variable in our data:
You are interested in what variables might be associated with obtaining a higher chance of getting a bonus (selling a house above $175,000). An association exists between two categorical variables if the distribution of one variable changes when the value of the other categorical changes. If there is no association, the distribution of the first variable is the same regardless of the value of the other variable. For example, if we wanted to know if obtaining a bonus on selling a house in Ames, Iowa was associated with whether the house had central air we could look at the distribution of bonus eligible houses. If we observe that 42% of homes with central air are bonus eligible and 42% of homes without central air are bonus eligible, then it appears that central air has no bearing on whether the home is bonus eligible. However, if instead we observe that only 3% of homes without central air are bonus eligible, but 44% of home with central air are bonus eligible, then it appears that having central air might be related to a home being bonus eligible.
To understand the distribution of categorical variables we need to look at frequency tables. A frequency table shows the number of observations that occur in certain categories or intervals. A one way frequency table examines all the categories of one variable. These are easily visualized with bar charts.
Let’s look at the distribution of both bonus eligibility and central air using the table
function. The ggplot
function with the geom_bar
function allows us to view our data in a bar chart.
##
## 0 1
## 1189 862
##
## N Y
## 130 1921
Frequency tables show single variables, but if we want to explore two variables together we look at cross-tabulation tables. A cross-tabulation table shows the number of observations for each combination of the row and column variables.
Let’s again examine bonus eligibility, but this time across levels of central air. Again, we can use the table
function. The prop.table
function allows us to compare two variables in terms of proportions instead of frequencies.
##
## 0 1
## N 126 4
## Y 1063 858
##
## 0 1
## N 0.061433447 0.001950268
## Y 0.518283764 0.418332521
From the above output we can see that 147 homes have no central air with only 5 of them being bonus eligible. However, there are 1904 homes that have central air with 835 of them being bonus eligible. For an even more detailed breakdown we can use the CrossTable
function.
##
##
## Cell Contents
## |-------------------------|
## | N |
## | Chi-square contribution |
## | N / Row Total |
## | N / Col Total |
## | N / Table Total |
## |-------------------------|
##
##
## Total Observations in Table: 2051
##
##
## | train$Bonus
## train$Central_Air | 0 | 1 | Row Total |
## ------------------|-----------|-----------|-----------|
## N | 126 | 4 | 130 |
## | 34.023 | 46.930 | |
## | 0.969 | 0.031 | 0.063 |
## | 0.106 | 0.005 | |
## | 0.061 | 0.002 | |
## ------------------|-----------|-----------|-----------|
## Y | 1063 | 858 | 1921 |
## | 2.302 | 3.176 | |
## | 0.553 | 0.447 | 0.937 |
## | 0.894 | 0.995 | |
## | 0.518 | 0.418 | |
## ------------------|-----------|-----------|-----------|
## Column Total | 1189 | 862 | 2051 |
## | 0.580 | 0.420 | |
## ------------------|-----------|-----------|-----------|
##
##
The advantage of the CrossTable
function is that we can easily get not only the frequencies, but the cell, row, and column proportions. For example, the third number in each cell gives us the row proportion. For homes without central air, 96.6% of them are not bonus eligible, while 3.4% of them are. For homes with central air, 56.1% of the homes are not bonus eligible, while 43.9% of them are. This would appear that the distribution of bonus eligible homes changes across levels of central air - a relationship between the two variables. This expected relationship needs to be tested statistically for verification.
7.1.1 Python Code
## Bonus
## 0 1162
## 1 889
## Name: count, dtype: int64
plot = (
ggplot(train)
+ geom_bar(aes(x='Bonus'))
+ labs(x='Bonus', y='Count')
+ theme_minimal()
)
plot.show()
## Central_Air
## Y 1913
## N 138
## Name: count, dtype: int64
plot = (
ggplot(train)
+ geom_bar(aes(x='Central_Air'))
+ labs(x='Central_Air', y='Count')
+ theme_minimal()
)
plot.show()
plot = (
ggplot(train)
+ geom_bar(aes(x='Bonus', fill='Central_Air'), position='stack')
+ labs(x='Bonus', y='Count', fill='Central_Air')
+ theme_minimal()
)
plot.show()
## Bonus 0 1
## Central_Air
## N 132 6
## Y 1030 883
7.2 Tests of Association
Much like in Chapter 2 we have statistical tests to evaluate relationships between two categorical variables. The null hypothesis for these statistical tests is that the two variables have no association - the distribution of one variable does not change across levels of another variable. The alternative hypothesis is an association between the two variables - the distribution of one variable changes across levels of another variable.
These statistical tests follow a \(\chi^2\)-distribution. The \(\chi^2\)-distribution is a distribution that has the following characteristics:
- Bounded below by 0
- Right-skewed
- One set of degrees of freedom
A plot of a variety of \(\chi^2\)-distributions is shown here:
Two common \(\chi^2\) tests are the Pearson and Likelihood Ratio \(\chi^2\) tests. They compare the observed count of observations in each cell of a cross-tabulation table between two variables to their expected count if there was no relationship. The expected cell count applies the overall distribution of one variable across all the levels of the other variable. For example, overall 59% of all homes are not bonus eligible. If that were to apply to every level of central air, then the 140 homes without central air would be expected to have 86.73 ( $ = 147 $ ) of them would be bonus eligible while 60.27 ( $ = 147 $ ) of them would not be bonus eligible. We actually observe 142 and 5 homes for each of these categories respectively. The further the observed data is from the expected data, the more evidence we have that there is a relationship between the two variables.
The test statistic for the Pearson \(\chi^2\) test is the following:
\[ \chi^2_P = \sum_{i=1}^R \sum_{j=1}^C \frac{(Obs_{i,j} - Exp_{i,j})^2}{Exp_{i,j}} \] From the equation above, the closer that the observed count of each cross-tabulation table cell to the expected count, the smaller the test statistic. As with all previous hypothesis tests, the smaller the test statistic, the larger the p-value, implying less evidence for the alternative hypothesis.
Let’s examine the relationship between central air and bonus eligibility using the chisq.test
function.
##
## Pearson's Chi-squared test with Yates' continuity correction
##
## data: table(train$Central_Air, train$Bonus)
## X-squared = 84.732, df = 1, p-value < 2.2e-16
The above results shows an extremely small p-value that is below any reasonable significance level. This implies that we have statistical evidence for a relationship between having central air and bonus eligibility of homes. The p-value comes from a \(\chi^2\)-distribution with degrees of freedom that equal the product of the number of rows minus one and the number of columns minus one.
Another common test is the Likelihood Ratio test. The test statistic for this is the following:
\[ \chi^2_L = 2 \times \sum_{i=1}^R \sum_{j=1}^C Obs_{i,j} \times \log(\frac{Obs_{i,j}}{Exp_{i,j}}) \]
The p-value comes from a \(\chi^2\)-distribution with degrees of freedom that equal the product of the number of rows minus one and the number of columns minus one. Both of the above tests have a sample size requirement. The sample size requirement is 80% or more of the cells in the cross-tabulation table need expected count larger than 5.
For smaller sample sizes, this might be hard to meet. In those situations, we can use a more computationally expensive test called Fisher’s exact test. This test calculates every possible permutation of the data being evaluated to calculate the p-value without any distributional assumptions. To perform this test we can use the fisher.test
function.
##
## Fisher's Exact Test for Count Data
##
## data: table(train$Central_Air, train$Bonus)
## p-value < 2.2e-16
## alternative hypothesis: true odds ratio is not equal to 1
## 95 percent confidence interval:
## 9.621024 95.281143
## sample estimates:
## odds ratio
## 25.40401
We see the same results as with the Pearson test because the assumptions were met for sample size.
Both the Pearson and Likelihood Ratio \(\chi^2\) tests can handle any type of categorical variable either ordinal, nominal, or both. However, ordinal variables provide us extra information since the order of the categories actually matters compared to nominal. We can test for even more with ordinal variables against other ordinal variables whether two ordinal variables have a linear relationship as compared to just a general one. An ordinal test for association is the Mantel-Haenszel \(\chi^2\) test. The test statistic for the Mantel-Haenszel \(\chi^2\) test is the following:
\[ \chi^2_{MH} = (n-1)r^2 \] where \(r^2\) is the Spearman’s correlation between the column and row variables. This test follows a \(\chi^2\)-distribution with only one degree of freedom.
Since both the central air and bonus eligibility variables are binary, they are ordinal. Since they are both ordinal, we should use the Mantel-Haenszel \(\chi^2\) test with the CMHtest
function. In the main output table, the first row is the Mantel-Haenszel \(\chi^2\) test.
## Chisq Df Prob
## 8.638875e+01 1.000000e+00 1.478232e-20
From here we can see another extremely small p-value as we saw in earlier, more general \(\chi^2\) tests.
7.2.1 Python Code
from scipy.stats import chi2_contingency
chi2_contingency(pd.crosstab(index = train['Central_Air'], columns = train['Bonus']), correction = True)
## Chi2ContingencyResult(statistic=89.93013829077994, pvalue=2.4671990961829127e-21, dof=1, expected_freq=array([[ 78.18430034, 59.81569966],
## [1083.81569966, 829.18430034]]))
from scipy.stats import fisher_exact
fisher_exact(pd.crosstab(index = train['Central_Air'], columns = train['Bonus']))
## SignificanceResult(statistic=18.860194174757282, pvalue=1.1286497199252816e-26)
No real Mantel-Haenszel options in Python that work for anything more than a 2x2 table so I wouldn’t trust them.
7.3 Measures of Association
Tests of association are best designed for just that, testing the existence of an association between two categorical variables. However, just like we saw in Chapter 1.1, hypothesis tests are impacted by sample size. When we have the same sample size, tests of association can rank significance of variables with p-values. However, when sample sizes are not the same between two tests, the tests of association are not best for comparing the strength of an association. In those scenarios, we have measures of strength of association that can be compared across any sample size.
Measures of association were not designed to test if an association exists, as that is what statistical testing is for. They are designed to measure the strength of association. There are dozens of these measures. Three of the most common are the following:
- Odds Ratios (only for comparing two binary variables)
- Cramer’s V (able to compare nominal variables with any number of categories)
- Spearman’s Correlation (able to compare ordinal variables with any number of categories)
An odds ratio indicates how much more likely, with respect to odds, a certain event occurs in one group relative to its occurrence in another group. The odds of an event occurring is not the same as the probability that an event occurs. The odds of an event occurring is the probability the event occurs divided by the probability that event does not occur.
\[ Odds = \frac{p}{1-p} \]
Let’s again examine the cross-tabulation table between central air and bonus eligibility.
##
##
## Cell Contents
## |-------------------------|
## | N |
## | Chi-square contribution |
## | N / Row Total |
## | N / Col Total |
## | N / Table Total |
## |-------------------------|
##
##
## Total Observations in Table: 2051
##
##
## | train$Bonus
## train$Central_Air | 0 | 1 | Row Total |
## ------------------|-----------|-----------|-----------|
## N | 126 | 4 | 130 |
## | 34.023 | 46.930 | |
## | 0.969 | 0.031 | 0.063 |
## | 0.106 | 0.005 | |
## | 0.061 | 0.002 | |
## ------------------|-----------|-----------|-----------|
## Y | 1063 | 858 | 1921 |
## | 2.302 | 3.176 | |
## | 0.553 | 0.447 | 0.937 |
## | 0.894 | 0.995 | |
## | 0.518 | 0.418 | |
## ------------------|-----------|-----------|-----------|
## Column Total | 1189 | 862 | 2051 |
## | 0.580 | 0.420 | |
## ------------------|-----------|-----------|-----------|
##
##
Let’s look at the row without central air. The probability that a home without central air is not bonus eligible is 96.6%. That implies that the odds of not being bonus eligible in homes without central air is 28.41 (= 0.966/0.034). For homes with central air, the odds of not being bonus eligible are 1.28 (= 0.561/0.439). The odds ratio between these two would be approximately 22.2 (= 28.41/1.28). In other words, homes without central air are 22.2 times more likely (in terms of odds) to not be bonus eligible as compared to homes with central air. This relationship is intuitive based on the numbers we have seen. Without going into details, it can also be shown that homes with central air are 22.2 times as likely (in terms of odds) to be bonus eligible.
We can use the OddsRatio
function to get these same results.
## [1] 25.42521
Cramer’s V is another measure of strength of association. Cramer’s V is calculated as follows:
\[ V = \sqrt{\frac{\chi^2_P/n}{\min(Rows-1, Columns-1)}} \]
Cramer’s V is bounded between 0 and 1 for every comparison other than two binary variables. For two binary variables being compared the bounds are -1 to 1. The idea is still the same for both. The further the value is from 0, the stronger the relationship. Unfortunately, unlike \(R^2\), Cramer’s V has no interpretative value. It can only be used for comparison.
We use the assocstats
function to get the Cramer’s V value. This function also provides the Pearson and Likelihood Ratio \(\chi^2\) tests as well.
## X^2 df P(> X^2)
## Likelihood Ratio 114.053 1 0
## Pearson 86.431 1 0
##
## Phi-Coefficient : 0.205
## Contingency Coeff.: 0.201
## Cramer's V : 0.205
Lastly, we have Spearman’s correlation. Much like the Mantel-Haenszel test of association was specifically designed for comparing two ordinal variables, Spearman correlation measures the strength of association between two ordinal variables. Spearman is not limited to only categorical data analysis as it was also seen back in Chapter 5 with detecting heteroskedasticity. Remember, Spearman correlation is a correlation on the ranks of the observations as compared to the actual values of the observations.
The cor.test
function that gave us Pearson’s correlation also provides Spearman’s correlation.
cor.test(x = as.numeric(ordered(train$Central_Air)),
y = as.numeric(ordered(train$Bonus)),
method = "spearman")
##
## Spearman's rank correlation rho
##
## data: x and y
## S = 1142769050, p-value < 2.2e-16
## alternative hypothesis: true rho is not equal to 0
## sample estimates:
## rho
## 0.2052824
As previously mentioned, these are only a few of the dozens of different measures of association that exist. However, they are the most used ones.
7.3.1 Python Code
Odds Ratios are the statistic calculated from the Fisher’s Exact test from the previous code:
from scipy.stats import fisher_exact
fisher_exact(pd.crosstab(index = train['Central_Air'], columns = train['Bonus']))
## SignificanceResult(statistic=18.860194174757282, pvalue=1.1286497199252816e-26)
from scipy.stats import fisher_exact
fisher_exact(pd.crosstab(index = train['Central_Air'], columns = train['Bonus']))
## SignificanceResult(statistic=18.860194174757282, pvalue=1.1286497199252816e-26)
from scipy.stats.contingency import association
association(pd.crosstab(index = train['Central_Air'], columns = train['Bonus']), method = "cramer")
## 0.2113604274410272
## SignificanceResult(statistic=0.21136042744102718, pvalue=3.822493169056582e-22)
7.4 Introduction to Logistic Regression
After exploring the categorical target variable, we can move on to modeling the categorical target variable. Logistic regression is a fundamental statistical analysis for data science and analytics. It part of a class of modeling techniques known as classification models since they are trying to predict categorical target variables. This target variable can be binary, ordinal, or even nominal in its structure. The primary focus will be binary logistic regression. It is the most common type of logistic regression, and sets up the foundation for both ordinal and nominal logistic regression.
Ordinary least squares regression is not the best approach to modeling categorical target variables. Mathematically, it can be shown that with a binary target variable coded as 0 and 1, an OLS linear regression model will produce the linear probability model.
7.4.1 Linear Probability Model
The linear probability model is not as widely used since probabilities do not tend to follow the properties of linearity in relation to their predictors. Also, the linear probability model possibly produces predictions outside of the bounds of 0 and 1 (where probabilities should be!). For completeness sake however, here is the linear probability model using the lm
function to try and predict bonus eligibility.
lp.model <- lm(Bonus ~ Gr_Liv_Area, data = train)
with(train, plot(x = Gr_Liv_Area, y = Bonus,
main = 'OLS Regression?',
xlab = 'Greater Living Area (Sqft)',
ylab = 'Bonus Eligibility'))
abline(lp.model)
Even though it doesn’t appear to really look like our data, let’s fit this linear probability model anyway for completeness sake.
##
## Call:
## lm(formula = Bonus ~ Gr_Liv_Area, data = train)
##
## Residuals:
## Min 1Q Median 3Q Max
## -1.48631 -0.29532 -0.09408 0.38524 0.86915
##
## Coefficients:
## Estimate Std. Error t value Pr(>|t|)
## (Intercept) -4.436e-01 2.782e-02 -15.95 <2e-16 ***
## Gr_Liv_Area 5.751e-04 1.756e-05 32.75 <2e-16 ***
## ---
## Signif. codes: 0 '***' 0.001 '**' 0.01 '*' 0.05 '.' 0.1 ' ' 1
##
## Residual standard error: 0.4001 on 2049 degrees of freedom
## Multiple R-squared: 0.3436, Adjusted R-squared: 0.3433
## F-statistic: 1072 on 1 and 2049 DF, p-value: < 2.2e-16
qqnorm(rstandard(lp.model),
ylab = "Standardized Residuals",
xlab = "Normal Scores",
main = "QQ-Plot of Residuals")
qqline(rstandard(lp.model))
plot(predict(lp.model), resid(lp.model),
ylab="Residuals", xlab="Predicted Values",
main="Residuals of Linear Probability Model")
abline(0, 0)
As we can see from the charts above, the assumptions of ordinary least squares don’t really hold in this situation. Therefore, we should be careful interpreting the results of the model. Maybe a better model won’t have these problems?
7.4.2 Binary Logistic Regression
Due to the limitations of the linear probability model, people typically just use the binary logistic regression model. The logistic regression model does not have the limitations of the linear probability model. The outcome of the logistic regression model is the probability of getting a 1 in a binary variable. That probability is calculated as follows:
\[ p_i = \frac{1}{1+e^{-(\beta_0 + \beta_1x_{1,i} + \cdots + \beta_k x_{k,i})}} \]
This function has the desired properties for predicting probabilities. The predicted probability from the above equation will always be between 0 and 1. The parameter estimates do not enter the function linearly (this is a non-linear regression model), and the rate of change of the probability varies as the predictor variables vary as seen in Figure 7.1.
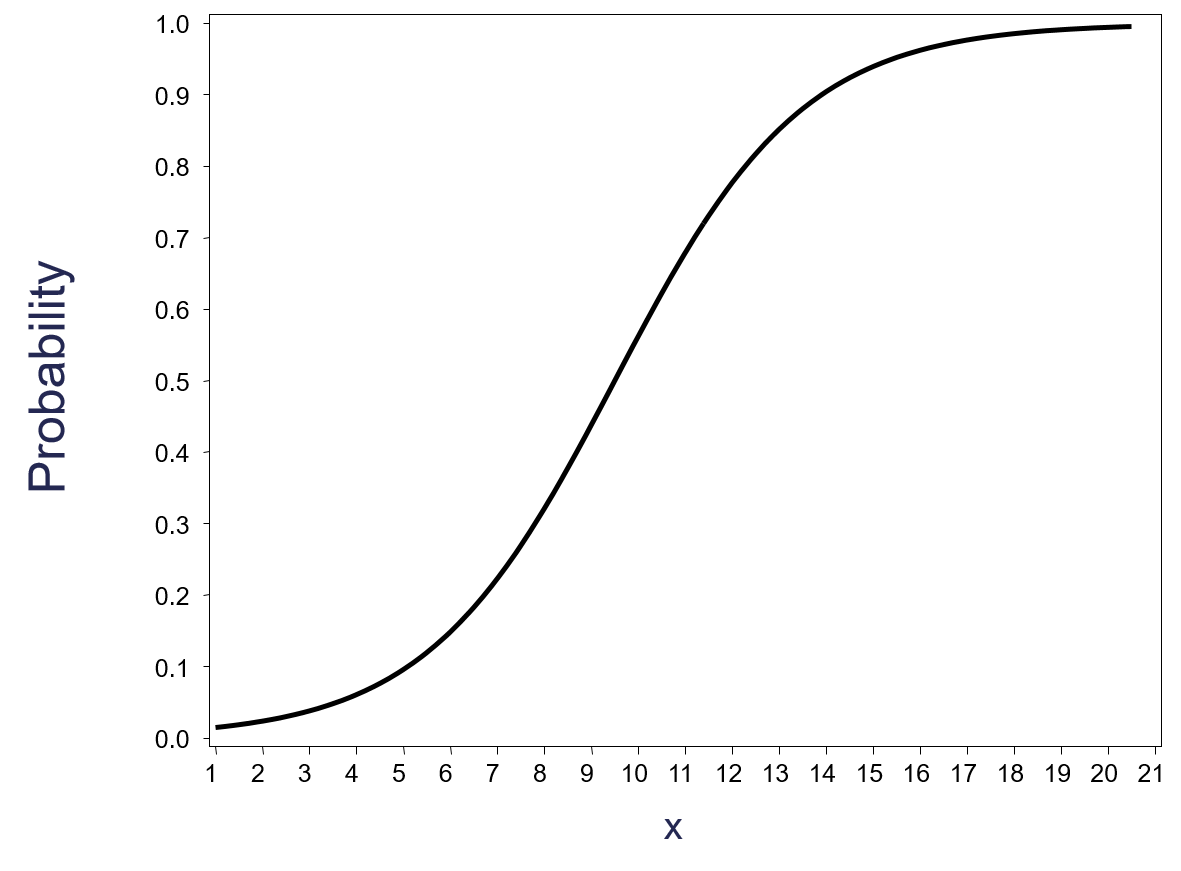
Figure 7.1: Example of a Logistic Curve
To create a linear model, a link function is applied to the probabilities. The specific link function for logistic regression is called the logit function.
\[ logit(p_i) = \log(\frac{p_i}{1-p_i}) = \beta_0 + \beta_1x_{1,i} + \cdots + \beta_k x_{k,i} \]
The relationship between the predictor variables and the logits are linear in nature as the logits themselves are unbounded. This structure looks much more like our linear regression model structure. However, logistic regression does not use OLS to estimate the coefficients in our model. OLS requires residuals which the logistic regression model does not provide. The target variable is binary in nature, but the predictions are probabilities. Therefore, we cannot calculate a traditional residual. Instead, logistic regression uses maximum likelihood estimation. This is not covered here.
There are two main assumptions for logistic regression:
- Independence of observations
- Linearity of the logit
The first assumption of independence is the same as we had for linear regression. The second assumption implies that the logistic function transformation (the logit) actually makes a linear relationship with our predictor variables. This assumption can be tested, but will not be covered in this brief introduction to logistic regression.
Let’s build a logistic regression model. We will use the glm
function to do this. The glm
function has a similar structure to the lm
function. The main difference is the family = binomial(link = "logit")
option to specify that we are uses a logistic regression model. Again, there are many different link functions, but only the logistic link function (the logit) is being used here.
ames_logit <- glm(Bonus ~ Gr_Liv_Area,
data = train, family = binomial(link = "logit"))
summary(ames_logit)
##
## Call:
## glm(formula = Bonus ~ Gr_Liv_Area, family = binomial(link = "logit"),
## data = train)
##
## Coefficients:
## Estimate Std. Error z value Pr(>|z|)
## (Intercept) -6.4980679 0.2908138 -22.34 <2e-16 ***
## Gr_Liv_Area 0.0041028 0.0001899 21.61 <2e-16 ***
## ---
## Signif. codes: 0 '***' 0.001 '**' 0.01 '*' 0.05 '.' 0.1 ' ' 1
##
## (Dispersion parameter for binomial family taken to be 1)
##
## Null deviance: 2790.9 on 2050 degrees of freedom
## Residual deviance: 1881.5 on 2049 degrees of freedom
## AIC: 1885.5
##
## Number of Fisher Scoring iterations: 5
Let’s examine the above output. Scanning down the output, you can see the actual logistic regression equation for the variable Gr_Liv_Area
. Here we can see that it appears to be a significant variable at predicting bonus eligibility. However, the coefficient reported does not have the same usable interpretation as in linear regression. An increase of one unit of greater living area square footage is linearly related to the logit not the probability of bonus eligibility. We can transform this coefficient to make it more interpretable. A single unit increase in greater living area square footage does have a \(100 \times (e^\hat{\beta}-1)\%\) increase in the average odds of bonus eligibility. We can use a combination of the exp
and coef
functions to obtain this number.
## Waiting for profiling to be done...
## 2.5 % 97.5 %
## (Intercept) -99.8493653 -99.9160362 -99.7373217
## Gr_Liv_Area 0.4111248 0.3746797 0.4494483
In other words, every additional square foot in greater living area in the home leads to an average increase in odds of 0.385% to be bonus eligible.
7.4.3 Adding Categorical Variables
Similar to linear regression as we learned in Chapter 3, logistic regression can have both continuous and categorical predictors for our categorical target variable. Let’s add both central air as well as number of fireplaces to our logistic regression model.
ames_logit2 <- glm(Bonus ~ Gr_Liv_Area + Central_Air + factor(Fireplaces),
data = train, family = binomial(link = "logit"))
summary(ames_logit2)
##
## Call:
## glm(formula = Bonus ~ Gr_Liv_Area + Central_Air + factor(Fireplaces),
## family = binomial(link = "logit"), data = train)
##
## Coefficients:
## Estimate Std. Error z value Pr(>|z|)
## (Intercept) -1.158e+01 7.855e-01 -14.742 < 2e-16 ***
## Gr_Liv_Area 4.166e-03 2.183e-04 19.087 < 2e-16 ***
## Central_AirY 4.583e+00 6.410e-01 7.150 8.69e-13 ***
## factor(Fireplaces)1 1.013e+00 1.281e-01 7.906 2.66e-15 ***
## factor(Fireplaces)2 3.649e-01 2.447e-01 1.491 0.136
## factor(Fireplaces)3 4.537e-01 1.041e+00 0.436 0.663
## ---
## Signif. codes: 0 '***' 0.001 '**' 0.01 '*' 0.05 '.' 0.1 ' ' 1
##
## (Dispersion parameter for binomial family taken to be 1)
##
## Null deviance: 2790.9 on 2050 degrees of freedom
## Residual deviance: 1679.1 on 2045 degrees of freedom
## AIC: 1691.1
##
## Number of Fisher Scoring iterations: 6
Just like with linear regression, categorical predictor variables are a comparison between two categories. Again, the coefficients from the logistic regression model need to be transformed to be interpreted.
## 2.5 % 97.5 %
## (Intercept) -99.9990646 -99.9998206 -99.9960225
## Gr_Liv_Area 0.4174825 0.3755847 0.4615482
## Central_AirY 9677.6456694 2981.4405074 39222.7811352
## factor(Fireplaces)1 175.3373539 114.3240763 254.2182994
## factor(Fireplaces)2 44.0359300 -11.0028029 132.5770593
## factor(Fireplaces)3 57.4104171 -77.5875200 1394.7564635
Let’s use the first fireplace variable as an example. A home with one fireplace has, on average, 167.04% higher odds of being bonus eligible as compared to a home with zero fireplaces.
7.4.4 Model Assessment
There are dozens of different ways to evaluate a logistic regression model. We will cover one popular way here - concordance. Counting the number of concordant, discordant, and tied pairs is a way to to assess how well the model fits the data.
To find concordant, discordant, and tied pairs, we must compare all of the 0’s in the target variable to all of the 1’s. For our example, we will compare every pair of homes where one home is bonus eligible and one is not (every 0 and 1 pair). A concordant pair is a 0 and 1 pair where the bonus eligible home (the 1 in our model) has a higher predicted probability than the non-bonus eligible home (the 0 in our model) - our model successfully ordered these two observations by probability. It does not matter what the actual predicted probability values are as long as the bonus eligible home has a higher predicted probability than the non-bonus eligible home. A discordant pair is a 0 and 1 pair where the bonus eligible home (the 1 in our model) has a lower predicted probability than the non-bonus eligible home (the 0 in our model) - our model unsuccessfully ordered the homes. It does not matter what the actual predicted probability values are as long as the bonus eligible home has a lower predicted probability than the non-bonus eligible home. A tied pair is a 0 and 1 pair where the bonus eligible home has the same predicted probability as the non-bonus eligible home - the model is confused and sees these two different things as the same. In general, you want a high percentage of concordant pairs and low percentages of discordant and tied pairs.
We can use the concordance
function from the survival
package to obtain these values on our predictions from the predict
function.
## Call:
## concordance.lm(object = ames_logit)
##
## n= 2051
## Concordance= 0.8675 se= 0.007596
## concordant discordant tied.x tied.y tied.xy
## 888831 135490 597 1075272 2085
From the above output we have a concordance of 86.3% for our model. There is no good or bad value as this can only be compared with another model to see which is better. Let’s compare this to our model with the categorical variables.
## Call:
## concordance.lm(object = ames_logit2)
##
## n= 2051
## Concordance= 0.8924 se= 0.006783
## concordant discordant tied.x tied.y tied.xy
## 914478 110174 266 1076141 1216
We can see that the model with categorical predictors added to it has a higher concordance at 88.4%. That implies that our model is correctly able to rank our observations 88.4% of the time. This is NOT the same thing as saying our model is 88.4% accurate. Accuracy (which is not covered here) deals with a prediction being correct or incorrect. Concordance is only measuring how often we are able to predict 1’s with higher probability than 0’s - again, correctly ranking the observations.
7.4.5 Variable Selection and Regularized Regression
As with linear regression in Chapters ?? and ??, logistic regression uses the same approaches to doing variable selection. In fact, the same function are used as well. Let’s use the step
function to apply a forward and backward selection to the logistic regression model.
train_sel_log <- train %>%
dplyr::select(Bonus,
Lot_Area,
Street,
Bldg_Type,
House_Style,
Overall_Qual,
Roof_Style,
Central_Air,
First_Flr_SF,
Second_Flr_SF,
Full_Bath,
Half_Bath,
Fireplaces,
Garage_Area,
Gr_Liv_Area,
TotRms_AbvGrd) %>%
mutate_if(is.numeric, ~replace_na(.,mean(., na.rm = TRUE)))
full.model <- glm(Bonus ~ . , data = train_sel_log)
empty.model <- glm(Bonus ~ 1, data = train_sel_log)
for.model <- step(empty.model,
scope = list(lower = formula(empty.model),
upper = formula(full.model)),
direction = "forward", k = log(dim(train_sel_log)[1]))
## Start: AIC=2934.01
## Bonus ~ 1
##
## Df Deviance AIC
## + Overall_Qual 9 236.49 1468.2
## + Full_Bath 1 320.70 2032.0
## + Gr_Liv_Area 1 328.02 2078.3
## + Garage_Area 1 357.99 2257.6
## + First_Flr_SF 1 389.04 2428.2
## + TotRms_AbvGrd 1 418.76 2579.2
## + Fireplaces 1 420.14 2585.9
## + House_Style 7 443.36 2742.0
## + Second_Flr_SF 1 464.15 2790.2
## + Half_Bath 1 465.56 2796.4
## + Lot_Area 1 474.57 2835.8
## + Central_Air 1 478.66 2853.3
## + Bldg_Type 4 481.65 2889.0
## <none> 499.72 2934.0
## + Street 1 499.23 2939.6
## + Roof_Style 5 492.33 2941.6
##
## Step: AIC=1468.19
## Bonus ~ Overall_Qual
##
## Df Deviance AIC
## + Gr_Liv_Area 1 208.62 1218.7
## + Full_Bath 1 208.66 1219.0
## + First_Flr_SF 1 216.99 1299.4
## + Lot_Area 1 221.01 1337.0
## + TotRms_AbvGrd 1 223.58 1360.7
## + Garage_Area 1 223.59 1360.8
## + Fireplaces 1 226.17 1384.3
## + Bldg_Type 4 229.34 1435.8
## + Second_Flr_SF 1 233.32 1448.2
## + Half_Bath 1 233.65 1451.1
## + House_Style 7 229.14 1456.8
## + Central_Air 1 234.84 1461.5
## <none> 236.49 1468.2
## + Street 1 236.36 1474.8
## + Roof_Style 5 235.77 1500.1
##
## Step: AIC=1218.69
## Bonus ~ Overall_Qual + Gr_Liv_Area
##
## Df Deviance AIC
## + Full_Bath 1 199.12 1130.7
## + House_Style 7 194.95 1133.1
## + First_Flr_SF 1 201.73 1157.4
## + Second_Flr_SF 1 202.48 1165.0
## + Garage_Area 1 202.58 1166.0
## + Lot_Area 1 202.94 1169.7
## + Fireplaces 1 206.04 1200.8
## + Central_Air 1 206.28 1203.2
## + Bldg_Type 4 205.04 1213.6
## + TotRms_AbvGrd 1 207.75 1217.7
## <none> 208.62 1218.7
## + Street 1 208.37 1223.9
## + Half_Bath 1 208.52 1225.3
## + Roof_Style 5 207.62 1246.9
##
## Step: AIC=1130.68
## Bonus ~ Overall_Qual + Gr_Liv_Area + Full_Bath
##
## Df Deviance AIC
## + House_Style 7 187.42 1059.9
## + First_Flr_SF 1 192.79 1072.1
## + Lot_Area 1 193.12 1075.5
## + Second_Flr_SF 1 193.47 1079.3
## + Garage_Area 1 194.00 1084.9
## + Fireplaces 1 195.10 1096.5
## + Bldg_Type 4 193.28 1100.1
## + Central_Air 1 196.72 1113.5
## + TotRms_AbvGrd 1 197.68 1123.4
## <none> 199.12 1130.7
## + Street 1 198.82 1135.2
## + Half_Bath 1 198.88 1135.8
## + Roof_Style 5 198.63 1163.7
##
## Step: AIC=1059.91
## Bonus ~ Overall_Qual + Gr_Liv_Area + Full_Bath + House_Style
##
## Df Deviance AIC
## + Lot_Area 1 183.49 1024.0
## + Bldg_Type 4 182.21 1032.6
## + Fireplaces 1 184.58 1036.2
## + Garage_Area 1 185.09 1041.8
## + Half_Bath 1 185.51 1046.5
## + Central_Air 1 186.12 1053.3
## + TotRms_AbvGrd 1 186.68 1059.4
## <none> 187.42 1059.9
## + First_Flr_SF 1 186.90 1061.9
## + Street 1 187.09 1064.0
## + Second_Flr_SF 1 187.17 1064.8
## + Roof_Style 5 186.32 1086.0
##
## Step: AIC=1024.03
## Bonus ~ Overall_Qual + Gr_Liv_Area + Full_Bath + House_Style +
## Lot_Area
##
## Df Deviance AIC
## + Fireplaces 1 181.35 1007.6
## + Bldg_Type 4 179.35 1007.8
## + Half_Bath 1 181.64 1010.9
## + Garage_Area 1 181.78 1012.5
## + Central_Air 1 182.34 1018.8
## + TotRms_AbvGrd 1 182.68 1022.5
## <none> 183.49 1024.0
## + First_Flr_SF 1 183.27 1029.2
## + Second_Flr_SF 1 183.41 1030.8
## + Street 1 183.48 1031.6
## + Roof_Style 5 182.40 1050.0
##
## Step: AIC=1007.63
## Bonus ~ Overall_Qual + Gr_Liv_Area + Full_Bath + House_Style +
## Lot_Area + Fireplaces
##
## Df Deviance AIC
## + Garage_Area 1 179.60 995.32
## + Half_Bath 1 179.69 996.33
## + Bldg_Type 4 177.71 996.50
## + Central_Air 1 180.43 1004.85
## + TotRms_AbvGrd 1 180.65 1007.28
## <none> 181.35 1007.63
## + First_Flr_SF 1 181.20 1013.56
## + Second_Flr_SF 1 181.31 1014.74
## + Street 1 181.34 1015.13
## + Roof_Style 5 180.17 1032.37
##
## Step: AIC=995.32
## Bonus ~ Overall_Qual + Gr_Liv_Area + Full_Bath + House_Style +
## Lot_Area + Fireplaces + Garage_Area
##
## Df Deviance AIC
## + Half_Bath 1 178.20 986.92
## + Bldg_Type 4 176.41 989.06
## <none> 179.60 995.32
## + TotRms_AbvGrd 1 178.99 995.95
## + Central_Air 1 179.05 996.71
## + First_Flr_SF 1 179.51 1001.92
## + Second_Flr_SF 1 179.58 1002.72
## + Street 1 179.59 1002.90
## + Roof_Style 5 178.37 1019.38
##
## Step: AIC=986.92
## Bonus ~ Overall_Qual + Gr_Liv_Area + Full_Bath + House_Style +
## Lot_Area + Fireplaces + Garage_Area + Half_Bath
##
## Df Deviance AIC
## + Bldg_Type 4 174.89 978.99
## <none> 178.20 986.92
## + TotRms_AbvGrd 1 177.65 988.17
## + Central_Air 1 177.85 990.54
## + First_Flr_SF 1 178.02 992.44
## + Second_Flr_SF 1 178.12 993.63
## + Street 1 178.20 994.51
## + Roof_Style 5 177.03 1011.59
##
## Step: AIC=978.99
## Bonus ~ Overall_Qual + Gr_Liv_Area + Full_Bath + House_Style +
## Lot_Area + Fireplaces + Garage_Area + Half_Bath + Bldg_Type
##
## Df Deviance AIC
## <none> 174.89 978.99
## + TotRms_AbvGrd 1 174.47 981.62
## + First_Flr_SF 1 174.52 982.27
## + Second_Flr_SF 1 174.69 984.30
## + Central_Air 1 174.81 985.62
## + Street 1 174.84 986.05
## + Roof_Style 5 173.70 1003.12
back.model <- step(full.model,
scope = list(lower = formula(empty.model),
upper = formula(full.model)),
direction = "backward", k = log(dim(train_sel_log)[1]))
## Start: AIC=1028.98
## Bonus ~ Lot_Area + Street + Bldg_Type + House_Style + Overall_Qual +
## Roof_Style + Central_Air + First_Flr_SF + Second_Flr_SF +
## Full_Bath + Half_Bath + Fireplaces + Garage_Area + Gr_Liv_Area +
## TotRms_AbvGrd
##
## Df Deviance AIC
## - Roof_Style 5 173.78 1004.0
## - Gr_Liv_Area 1 172.68 1021.5
## - Central_Air 1 172.71 1021.9
## - Street 1 172.73 1022.1
## - Second_Flr_SF 1 172.82 1023.2
## - First_Flr_SF 1 173.01 1025.5
## - TotRms_AbvGrd 1 173.03 1025.7
## <none> 172.66 1029.0
## - House_Style 7 177.41 1031.2
## - Garage_Area 1 173.50 1031.2
## - Lot_Area 1 173.90 1036.0
## - Bldg_Type 4 175.97 1037.4
## - Half_Bath 1 174.09 1038.2
## - Fireplaces 1 174.10 1038.4
## - Full_Bath 1 183.82 1149.8
## - Overall_Qual 9 215.71 1416.8
##
## Step: AIC=1003.99
## Bonus ~ Lot_Area + Street + Bldg_Type + House_Style + Overall_Qual +
## Central_Air + First_Flr_SF + Second_Flr_SF + Full_Bath +
## Half_Bath + Fireplaces + Garage_Area + Gr_Liv_Area + TotRms_AbvGrd
##
## Df Deviance AIC
## - Gr_Liv_Area 1 173.79 996.58
## - Street 1 173.83 996.99
## - Central_Air 1 173.86 997.38
## - Second_Flr_SF 1 173.93 998.24
## - First_Flr_SF 1 174.12 1000.40
## - TotRms_AbvGrd 1 174.21 1001.50
## - House_Style 7 178.28 1003.11
## <none> 173.78 1003.99
## - Garage_Area 1 174.53 1005.27
## - Bldg_Type 4 177.00 1011.23
## - Lot_Area 1 175.08 1011.70
## - Fireplaces 1 175.15 1012.51
## - Half_Bath 1 175.23 1013.51
## - Full_Bath 1 185.72 1132.74
## - Overall_Qual 9 217.19 1392.75
##
## Step: AIC=996.58
## Bonus ~ Lot_Area + Street + Bldg_Type + House_Style + Overall_Qual +
## Central_Air + First_Flr_SF + Second_Flr_SF + Full_Bath +
## Half_Bath + Fireplaces + Garage_Area + TotRms_AbvGrd
##
## Df Deviance AIC
## - Street 1 173.85 989.59
## - Central_Air 1 173.88 989.97
## - TotRms_AbvGrd 1 174.26 994.42
## <none> 173.79 996.58
## - House_Style 7 178.40 996.80
## - Garage_Area 1 174.55 997.86
## - Second_Flr_SF 1 174.55 997.89
## - Bldg_Type 4 177.01 1003.66
## - Lot_Area 1 175.10 1004.27
## - Fireplaces 1 175.16 1005.08
## - Half_Bath 1 175.25 1006.08
## - First_Flr_SF 1 177.59 1033.29
## - Full_Bath 1 185.73 1125.21
## - Overall_Qual 9 217.28 1385.94
##
## Step: AIC=989.59
## Bonus ~ Lot_Area + Bldg_Type + House_Style + Overall_Qual + Central_Air +
## First_Flr_SF + Second_Flr_SF + Full_Bath + Half_Bath + Fireplaces +
## Garage_Area + TotRms_AbvGrd
##
## Df Deviance AIC
## - Central_Air 1 173.93 982.89
## - TotRms_AbvGrd 1 174.32 987.55
## <none> 173.85 989.59
## - House_Style 7 178.45 989.78
## - Second_Flr_SF 1 174.59 990.77
## - Garage_Area 1 174.63 991.15
## - Bldg_Type 4 177.01 996.11
## - Fireplaces 1 175.22 998.09
## - Half_Bath 1 175.31 999.09
## - Lot_Area 1 175.38 999.93
## - First_Flr_SF 1 177.60 1025.76
## - Full_Bath 1 185.75 1117.81
## - Overall_Qual 9 217.39 1379.42
##
## Step: AIC=982.89
## Bonus ~ Lot_Area + Bldg_Type + House_Style + Overall_Qual + First_Flr_SF +
## Second_Flr_SF + Full_Bath + Half_Bath + Fireplaces + Garage_Area +
## TotRms_AbvGrd
##
## Df Deviance AIC
## - TotRms_AbvGrd 1 174.41 980.93
## <none> 173.93 982.89
## - House_Style 7 178.57 983.56
## - Second_Flr_SF 1 174.66 983.96
## - Garage_Area 1 174.80 985.55
## - Fireplaces 1 175.33 991.77
## - Bldg_Type 4 177.30 991.85
## - Lot_Area 1 175.48 993.54
## - Half_Bath 1 175.49 993.60
## - First_Flr_SF 1 177.66 1018.82
## - Full_Bath 1 185.99 1112.84
## - Overall_Qual 9 217.41 1371.92
##
## Step: AIC=980.93
## Bonus ~ Lot_Area + Bldg_Type + House_Style + Overall_Qual + First_Flr_SF +
## Second_Flr_SF + Full_Bath + Half_Bath + Fireplaces + Garage_Area
##
## Df Deviance AIC
## - Second_Flr_SF 1 174.84 978.44
## <none> 174.41 980.93
## - House_Style 7 179.11 982.16
## - Garage_Area 1 175.35 984.33
## - Fireplaces 1 175.81 989.79
## - Bldg_Type 4 177.90 991.08
## - Half_Bath 1 176.01 992.06
## - Lot_Area 1 176.04 992.41
## - First_Flr_SF 1 177.80 1012.82
## - Full_Bath 1 186.17 1107.15
## - Overall_Qual 9 218.40 1373.68
##
## Step: AIC=978.44
## Bonus ~ Lot_Area + Bldg_Type + House_Style + Overall_Qual + First_Flr_SF +
## Full_Bath + Half_Bath + Fireplaces + Garage_Area
##
## Df Deviance AIC
## - House_Style 7 179.16 975.13
## <none> 174.84 978.44
## - Garage_Area 1 175.84 982.42
## - Fireplaces 1 176.50 990.21
## - Lot_Area 1 176.51 990.26
## - Bldg_Type 4 178.55 990.93
## - Half_Bath 1 176.88 994.52
## - First_Flr_SF 1 177.98 1007.28
## - Full_Bath 1 189.54 1136.37
## - Overall_Qual 9 218.94 1371.07
##
## Step: AIC=975.13
## Bonus ~ Lot_Area + Bldg_Type + Overall_Qual + First_Flr_SF +
## Full_Bath + Half_Bath + Fireplaces + Garage_Area
##
## Df Deviance AIC
## <none> 179.16 975.13
## - Garage_Area 1 180.54 983.22
## - Fireplaces 1 180.54 983.25
## - Bldg_Type 4 182.71 984.80
## - Lot_Area 1 180.78 985.91
## - Half_Bath 1 183.35 1014.88
## - First_Flr_SF 1 183.82 1020.09
## - Full_Bath 1 198.19 1174.50
## - Overall_Qual 9 225.96 1382.45
In the above two approaches we used the BIC selection criteria. Here both forward and backward selection actually picked the same model. Let’s check the concordance of this model.
## Call:
## concordance.lm(object = back.model)
##
## n= 2051
## Concordance= 0.9608 se= 0.003626
## concordant discordant tied.x tied.y tied.xy
## 984770 40147 1 1077327 30
Not surprisingly, this model outperforms the previous model that we had with a concordance of 96.1%.
Although not covered in detail here, regularized regression can also be applied to logistic regression to get a different view. This might be helpful with the multicollinearity present in these predictor variables. Again, we can use the glmnet
function with the addition of a family = "binomial"
option.
7.4.6 Python Code
Linear Probability Model
import statsmodels.formula.api as smf
lp_model = smf.ols("Bonus ~ Gr_Liv_Area", data = train).fit()
lp_model.summary()
Dep. Variable: | Bonus | R-squared: | 0.328 |
---|---|---|---|
Model: | OLS | Adj. R-squared: | 0.327 |
Method: | Least Squares | F-statistic: | 998.6 |
Date: | Tue, 22 Jul 2025 | Prob (F-statistic): | 7.04e-179 |
Time: | 08:47:49 | Log-Likelihood: | -1063.1 |
No. Observations: | 2051 | AIC: | 2130. |
Df Residuals: | 2049 | BIC: | 2142. |
Df Model: | 1 | ||
Covariance Type: | nonrobust |
coef | std err | t | P>|t| | [0.025 | 0.975] | |
---|---|---|---|---|---|---|
Intercept | -0.4056 | 0.028 | -14.471 | 0.000 | -0.461 | -0.351 |
Gr_Liv_Area | 0.0006 | 1.76e-05 | 31.601 | 0.000 | 0.001 | 0.001 |
Omnibus: | 0.074 | Durbin-Watson: | 2.088 |
---|---|---|---|
Prob(Omnibus): | 0.964 | Jarque-Bera (JB): | 0.093 |
Skew: | 0.014 | Prob(JB): | 0.955 |
Kurtosis: | 2.983 | Cond. No. | 4.96e+03 |
Notes:
[1] Standard Errors assume that the covariance matrix of the errors is correctly specified.
[2] The condition number is large, 4.96e+03. This might indicate that there are
strong multicollinearity or other numerical problems.
train['pred_lp'] = lp_model.predict()
train['resid_lp'] = lp_model.resid
train[['Bonus', 'pred_lp', 'resid_lp']].head(n = 10)
## Bonus pred_lp resid_lp
## 2278 0 0.727900 -0.727900
## 1379 0 0.098187 -0.098187
## 2182 0 0.191808 -0.191808
## 1436 1 0.515023 0.484977
## 1599 0 0.202954 -0.202954
## 452 1 0.322209 0.677791
## 585 1 1.065604 -0.065604
## 1078 0 0.500534 -0.500534
## 2452 1 1.002075 -0.002075
## 1658 0 0.565735 -0.565735
plt.cla()
ax = sns.relplot(data = train, y = "resid_lp", x = "pred_lp")
ax.set(ylabel = 'Residuals',
xlabel = 'Predicted Probability of Bonus')
Binary Logistic Regression
## Optimization terminated successfully.
## Current function value: 0.466728
## Iterations 7
Dep. Variable: | Bonus | No. Observations: | 2051 |
---|---|---|---|
Model: | Logit | Df Residuals: | 2049 |
Method: | MLE | Df Model: | 1 |
Date: | Tue, 22 Jul 2025 | Pseudo R-squ.: | 0.3179 |
Time: | 08:47:51 | Log-Likelihood: | -957.26 |
converged: | True | LL-Null: | -1403.4 |
Covariance Type: | nonrobust | LLR p-value: | 4.571e-196 |
coef | std err | z | P>|z| | [0.025 | 0.975] | |
---|---|---|---|---|---|---|
Intercept | -6.3503 | 0.286 | -22.231 | 0.000 | -6.910 | -5.790 |
Gr_Liv_Area | 0.0041 | 0.000 | 21.645 | 0.000 | 0.004 | 0.004 |
## Intercept -99.825386
## Gr_Liv_Area 0.405848
## dtype: float64
Adding Categorical Variables
## Warning: Maximum number of iterations has been exceeded.
## Current function value: 0.421157
## Iterations: 35
## C:\PROGRA~3\ANACON~1\Lib\site-packages\statsmodels\base\model.py:607: ConvergenceWarning: Maximum Likelihood optimization failed to converge. Check mle_retvals
Dep. Variable: | Bonus | No. Observations: | 2051 |
---|---|---|---|
Model: | Logit | Df Residuals: | 2044 |
Method: | MLE | Df Model: | 6 |
Date: | Tue, 22 Jul 2025 | Pseudo R-squ.: | 0.3845 |
Time: | 08:47:51 | Log-Likelihood: | -863.79 |
converged: | False | LL-Null: | -1403.4 |
Covariance Type: | nonrobust | LLR p-value: | 6.407e-230 |
coef | std err | z | P>|z| | [0.025 | 0.975] | |
---|---|---|---|---|---|---|
Intercept | -10.1177 | 0.667 | -15.176 | 0.000 | -11.424 | -8.811 |
C(Central_Air)[T.Y] | 3.4809 | 0.536 | 6.495 | 0.000 | 2.430 | 4.531 |
C(Fireplaces)[T.1] | 1.1059 | 0.126 | 8.766 | 0.000 | 0.859 | 1.353 |
C(Fireplaces)[T.2] | 0.6891 | 0.235 | 2.930 | 0.003 | 0.228 | 1.150 |
C(Fireplaces)[T.3] | -0.4379 | 0.964 | -0.454 | 0.650 | -2.328 | 1.452 |
C(Fireplaces)[T.4] | 17.4289 | 3.95e+04 | 0.000 | 1.000 | -7.73e+04 | 7.73e+04 |
Gr_Liv_Area | 0.0039 | 0.000 | 18.786 | 0.000 | 0.004 | 0.004 |
## Intercept -9.999596e+01
## C(Central_Air)[T.Y] 3.149026e+03
## C(Fireplaces)[T.1] 2.021892e+02
## C(Fireplaces)[T.2] 9.919188e+01
## C(Fireplaces)[T.3] -3.545996e+01
## C(Fireplaces)[T.4] 3.709002e+09
## Gr_Liv_Area 3.928177e-01
## dtype: float64
## Intercept 4.035898e-05
## C(Central_Air)[T.Y] 3.249026e+01
## C(Fireplaces)[T.1] 3.021892e+00
## C(Fireplaces)[T.2] 1.991919e+00
## C(Fireplaces)[T.3] 6.454004e-01
## C(Fireplaces)[T.4] 3.709002e+07
## Gr_Liv_Area 1.003928e+00
## dtype: float64
Model Assessment
Python doesn’t have concordant / discordant pair calculations. We will learn in Fall semester other metrics to evaluate a logistic regression model that Python does have.
Variable Selection and Regularized Regression
Python does NOT have nice capabilities to do variable selection automatically in statsmodels, scikitlearn, or scipy. All resources I can find involve downloading and installing a package (mlxtend) that is not included by default in anaconda or writing your own function. Scikit learn has something similar but uses the model’s coefficients (!!!) to select, not p-values. Scikit learn can do this by evaluating a metric on cross-validation, but that is not covered until machine learning in Fall 3.